26 Jun 2011
Since I started using RX, events have played less and less of a role in my code. It’s true that in the same way LINQ has relegated for-loops to only niche situations, RX is making events all but obsolete. What does this mean for the EventAggregator
? Certainly, you can implement your own version using RX. But, if you happen to be using MEF (preferably as your IOC container) you can even get rid of that.
Introducing the Streams Pattern
First we need to create a “stream provider”. The purpose of this class is to establish a single place that “owns” the stream. I like to use the stream provider to establish default values and behavior:
public static class StreamNames
{
private const string Prefix = "Streams_";
public const string Accounts = Prefix + "Accounts";
public const string SelectedAccount = Prefix + "SelectedAccount";
}
// No need to export this type. An instance will be
// shared between the two child exports.
public class AccountStreamProvider
{
[ImportingConstructor]
public AccountStreamProvider(IAccountService accountService)
{
// Defer the query for the list of accounts until the first subscription
var publishedAccounts = Observable
.Defer(() => Observable.Return(accountService.GetAccounts()))
.Publish();
publishedAccounts.Connect();
Accounts = publishedAccounts;
SelectedAccount = new ReplaySubject(1);
// Take the first account and set it as the initially selected account.
Accounts
.Take(1)
.Select(Enumerable.FirstOrDefault)
.Subscribe(SelectedAccount.OnNext);
}
[Export(StreamNames.Accounts)]
public IObservable<IEnumerable<Account>> Accounts { get; set; }
[Export(StreamNames.SelectedAccount)]
[Export(StreamNames.SelectedAccount, typeof(IObservable<Account>)]
public ISubject<Account> SelectedAccount { get;set; }
}
Already the benefits of RX over the EventAggregator
are showing.
Now we just need to get a reference to our exports in a relevant view model:
public static class Extensions
{
public static void DisposeWith(this IDisposable source, CompositeDisposable disposables)
{
disposables.Add(source);
}
}
[Export, PartCreationPolicty(CreationPolicy.NotShared)]
public class AccountViewModel : BindableBase, IDisposable
{
private readonly Streams _streams;
private readonly CompositeDisposable _disposables;
[Export]
public class Streams
{
[Import(StreamNames.Accounts)]
public IObservable<IEnumerable<Account>> Accounts { get; set; }
[Import(StreamNames.SelectedAccount)]
public ISubject<Account> SelectedAccount { get; set; }
}
[ImportingConstructor]
public AccountViewModel(Streams streams)
{
_streams = streams;
_disposables = new CompositeDisposable();
_streams
.Accounts
.Subscribe(a => Accounts = a)
.DisposeWith(_disposables);
_streams
.SelectedAccount
.Subscribe(a => SelectedAccount = a)
.DisposeWith(_disposables);
}
private IEnumerable<Account> _accounts;
public IEnumerable<Account> Accounts
{
get { return _accounts; }
private set { SetProperty(ref _accounts, value, "Accounts"); }
}
private Account _selectedAccount;
public Account SelectedAccount
{
get { return _selectedAcccount; }
private set { SetProperty(ref _selectedAccount, value, "SelectedAccount", OnSelectedAccountChanged); }
}
// This method is only called when _selectedAccount
// actually changes, so there's no indirect recursion.
private void OnSelectedAccountChanged()
{
_streams.SelectedAccount.OnNext(_selectedAccount);
}
public void Dispose()
{
_disposables.Dispose();
}
}
By the way, I’m using BinableBase
from my INPC template.
Introducing the Config Pattern
If you’ve been using RX for a while, you might have noticed that I forgot to put my setters on the dispatcher. Sure, I could rely on automatic dispatching to fix that problem, but if my queries got any more complicated It’d be better for me to take care of the dispatch myself.
Of course, the problem with ObserveOnDispatcher
is that it makes testing a huge pain. Fortunately, we can use MEF to get around that problem, too.
public class AccountViewModel : BindableBase, IDisposable
{
private readonly Streams _streams;
private readonly Config _config;
private readonly CompositeDisposable _disposables;
[Export]
public class Streams
{
[Import(StreamNames.Accounts)]
public IObservable<IEnumerable<Account>> Accounts { get; set; }
[Import(StreamNames.SelectedAccount)]
public ISubject<Account> SelectedAccount { get; set; }
}
[Export]
public class Config
{
public virtual IScheduler DispatcherScheduler { get { return Scheduler.Dispatcher; } }
}
[ImportingConstructor]
public AccountViewModel(Streams streams, Config config)
{
_streams = streams;
_config = config;
_disposables = new CompositeDisposable();
_streams
.Accounts
.ObserveOn(_config.DispatcherScheduler)
.Subscribe(a => Accounts = a )
.DisposeWith(_disposables);
_streams
.SelectedAccount
.ObserveOn(_config.DispatcherScheduler)
.Subscribe(a => SelectedAccount = a)
.DisposeWith(_disposables);
}
// ...
}
Since the DispatcherScheduler
property is virtual, it’s easy to mock it out. Using Moq, all you need to do is:
Mock.Of<AccountViewModel.Config>(m => m.DispatcherScheduler == Scheduler.Immediate)
08 May 2011
Perhaps one of the most ambivalent things about putting code on GitHub is that it’s more or less an open project. It’s great that people (including yourself) can continue to work on it, but it seems to lack closure so that you can move on with your life.
So one of the things that I’ve been missing in my BindableBase
class is property coercion, a la dependency properties. It’s a pretty smart idea; you can keep values in a valid state without pushing change notification. Unfortunately, there are some problems that crop up pretty quickly in INotifyPropertyChanged
based view models.
Consider a Foo property that refuses to set a value less than zero.
private int _foo;
public int Foo
{
get { return _foo; }
set
{
if(_foo >= 0)
SetProperty(ref _foo, value, "Foo");
}
}
That looks like pretty good coercion, but the problem is that your binding and your property are now out of sync. That is, the binding told your object to set a value and assumed it did; you gave it no notification to the contrary. So while your object retains the old value, the bound TextBox
will blissfully report -23.
A more brute force option would raise PropertyChanged
in the event of this kind of coercion, but that breaks the code contract for INotifyPropertyChanged
in that nothing changed. So how do we get around this problem?
If you take a look at the invocation list of your PropertyChanged
event with some bound variables, you’ll notice that there’s a PropertyChangedEventManager
hooked up.
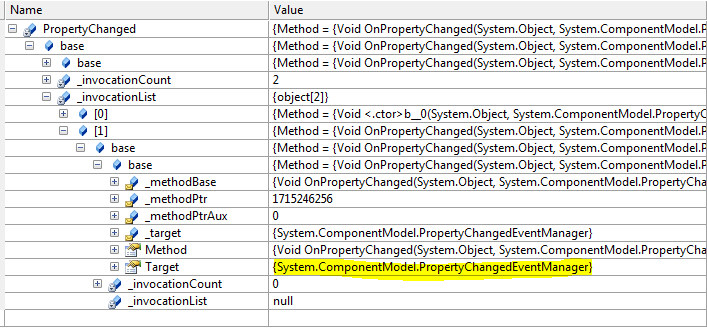
Considering that the other item in the list is my default delegate, this object must be responsible for communicating my events to the binding system
Of course, the next thing to do is fire up Reflector and take a look at what’s there.
public class PropertyChangedEventManager : WeakEventManager
{
// Fields
private WeakEventManager.ListenerList _proposedAllListenersList;
private static readonly string AllListenersKey;
// Methods
static PropertyChangedEventManager();
private PropertyChangedEventManager();
public static void AddListener(INotifyPropertyChanged source, IWeakEventListener listener, string propertyName);
private void OnPropertyChanged(object sender, PropertyChangedEventArgs args);
private void PrivateAddListener(INotifyPropertyChanged source, IWeakEventListener listener, string propertyName);
private void PrivateRemoveListener(INotifyPropertyChanged source, IWeakEventListener listener, string propertyName);
protected override bool Purge(object source, object data, bool purgeAll);
public static void RemoveListener(INotifyPropertyChanged source, IWeakEventListener listener, string propertyName);
protected override void StartListening(object source);
protected override void StopListening(object source);
// Properties
private static PropertyChangedEventManager CurrentManager { get; }
}
Basically, AddListener
access the private CurrentManager
singleton and subscribes the given listener to OnPropertyChanged
. Fortunately for us, there’s a flaw in th the implementation of OnPropertyChanged
. What it does is it gets the list of listeners based on the sender and raises their event with the given sender and args. The problem here is that it doesn’t verify that the object raising the event is actually the sender! That is to say, we should be able to send a fake PropertyChanged event through another object acting as a surrogate. All we need to do is add that object to the PropertyChangedEvenManager’s list and start impersonating.
To that end, I added this private class to BindableBase
to lazily add the INotifyPropertyChanged
proxy object to the PropertyChangedEventManager
. Since we’re not actually interested in the events, I made a stub implementation of IWeakEventListener
that returns false constantly to indicate it’s not handling the event. Finally, I hold onto both of these references to keep them from being garbage collected.
private class PropertyChangedEventManagerProxy
{
// We need to hold on to these refs to keep it from getting GC'd
private readonly NotifyPropertyChangedProxy _notifyPropertyChangedProxy;
private readonly IWeakEventListener _weakEventListener;
private PropertyChangedEventManagerProxy()
{
_notifyPropertyChangedProxy = new NotifyPropertyChangedProxy();
_weakEventListener = new WeakListenerStub();
PropertyChangedEventManager.AddListener(_notifyPropertyChangedProxy, _weakEventListener, string.Empty);
}
public void RaisePropertyChanged(object sender, string propertyName)
{
_notifyPropertyChangedProxy.Raise(sender, new PropertyChangedEventArgs(propertyName));
}
private static PropertyChangedEventManagerProxy _instance;
public static PropertyChangedEventManagerProxy Instance { get { return _instance ?? (_instance = new PropertyChangedEventManagerProxy()); } }
private class NotifyPropertyChangedProxy : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged = delegate { };
public void Raise(object sender, PropertyChangedEventArgs e)
{
PropertyChanged(sender, e);
}
}
private class WeakListenerStub : IWeakEventListener
{
public bool ReceiveWeakEvent(Type managerType, object sender, EventArgs e) { return false; }
}
}
The only thing left to do is add the coerce value function as an optional parameter on SetProperty
and hook it up:
protected void SetProperty<T>(
ref T backingStore,
T value,
string propertyName,
Action onChanged = null,
Action<T> onChanging = null,
Func<T, T> coerceValue = null)
{
VerifyCallerIsProperty(propertyName);
var effectiveValue = coerceValue != null ? coerceValue(value) : value;
if (EqualityComparer<T>.Default.Equals(backingStore, effectiveValue))
{
// If we coerced this value and the coerced value is not equal to the original, we need to
// send a fake PropertyChanged event to notify WPF that this value isn't what it thinks it is.
if (coerceValue != null && !EqualityComparer<T>.Default.Equals(value, effectiveValue))
PropertyChangedEventManagerProxy.Instance.RaisePropertyChanged(this, propertyName);
return;
}
if (onChanging != null) onChanging(effectiveValue);
OnPropertyChanging(propertyName);
backingStore = effectiveValue;
if (onChanged != null) onChanged();
OnPropertyChanged(propertyName);
}
And there you have it. All the benefits of dependency property coercion, and the same short, sweet SetProperty
syntax.
As before, everything is on GitHub so you can take a look at the whole thing and fork it if you see something to be improved.
20 Feb 2011
It’s sad how much controversy there is in doing something as simple as raising property change notification to our subscribers. It seems to me that we should have settled on something by now and moved on to bigger problems, yet, still, I see developers at every level of experience doing it differently.
I want to inform you all that you’re doing it wrong.
The Problem
Most projects choose to implement INotifyPropertyChanged
instead of extending DependencyObject
for the sole reason that dependency properties are an enormous pain to write. Having worked on a large project that used the latter approach, I’ve entirely sworn it off, and I don’t care what kind of tooling you say makes life better. It’s just too complicated. On top of that, it’s really difficult to subscribe to changes programmatically. Sure you can go through DependencyPropertyDescriptor
and subscribe yourself, but that’s a hell of a lot harder than +=
. So that leaves some people with properties that look like this:
private int _myValue;
public int MyValue
{
get { return _myValue; }
set { _myValue = value; OnPropertyChanged("MyValue"); }
}
Not bad, perhaps. It just doesn’t do much. What about INotifyPropertyChanging
? How do I do things internally when that property changes other than subscribing to my own PropertyChanged
event?
These things could almost be overlooked. The real kicker is that this isn’t actually a proper implementation of INotifyPropertyChanged
. According to MSDN, the PropertyChanged
event is described as, “Occurs when a property value changes,” and this code will raise an event even if the value doesn’t. Their implementation looks like this:
private string customerNameValue = String.Empty;
public string CustomerName
{
get
{
return this.customerNameValue;
}
set
{
if (value != this.customerNameValue)
{
this.customerNameValue = value;
NotifyPropertyChanged("CustomerName");
}
}
}
Aside from their code style (or lack thereof), allow me to point out a few things. First – and foremost – they’re comparing string equality with operators, no mere venial sin in my book. Secondly, this puts the onus of figuring out how to compare two types on the property writer ad-hoc, rather than using something more intelligent. Finally, does anyone else think that 17 lines is a few too many for a dirt simple property? It’s crazy.
The Trouble with Lambda
Some very smart programmers I know like to have their NotifyPropertyChanged
method take an Expression<Func> so you can do something like:
private int _myValue;
public int MyValue
{
get { return _myValue; }
set { _myValue = value; NotifyPropertyChanged(() => MyValue); }
}
They say it improves type checking and the ease of refactoring. But as it turns out, it’s god-awful for performance, which can run you into a lot of problems if you don’t keep track of even moderate update bursts.
I’d almost be willing to accept the performance trade-off if it weren’t for the fact that the only place that’s calling it is inside the property setter. When you’re refactoring, I don’t see it as too much effort to go ahead and fix the string in the underlying property setter while you’re there; you have to fix the backing member anyway.
Really what we need is lambdas for subscription. All those places out in your code that subscribe to PropertyChanged
and then you check the property name with a string! That’s the real refactoring nightmare. What I want to do is myViewModel.SubscribeToPropertyChanged(vm => vm.Foo, OnFooChanged);
The implementation isn’t that complex at all:
public static IDisposable SubscribeToPropertyChanged<TSource, TProp>(
this TSource source,
Expression<Func<TSource, TProp>> propertySelector,
Action onChanged)
where TSource : INotifyPropertyChanged
{
if (source == null) throw new ArgumentNullException("source");
if (propertySelector == null) throw new ArgumentNullException("propertySelector");
if (onChanged == null) throw new ArgumentNullException("onChanged");
var subscribedPropertyName = GetPropertyName(propertySelector);
PropertyChangedEventHandler handler = (s, e) =>
{
if (string.Equals(e.PropertyName, subscribedPropertyName, StringComparison.InvariantCulture))
onChanged();
};
source.PropertyChanged += handler;
return Disposable.Create(() => source.PropertyChanged -= handler);
}
public static IDisposable SubscribeToPropertyChanging<TSource, TProp>(
this TSource source, Expression<Func<TSource,
TProp>> propertySelector,
Action onChanging)
where TSource : INotifyPropertyChanging
{
if (source == null) throw new ArgumentNullException("source");
if (propertySelector == null) throw new ArgumentNullException("propertySelector");
if (onChanging == null) throw new ArgumentNullException("onChanged");
var subscribedPropertyName = GetPropertyName(propertySelector);
PropertyChangingEventHandler handler = (s, e) =>
{
if (string.Equals(e.PropertyName, subscribedPropertyName, StringComparison.InvariantCulture))
onChanging();
};
source.PropertyChanging += handler;
return Disposable.Create(() => source.PropertyChanging -= handler);
}
private static string GetPropertyName<TSource, TProp>(Expression<Func<TSource, TProp>> propertySelector)
{
var memberExpr = propertySelector.Body as MemberExpression;
if (memberExpr == null) throw new ArgumentException("must be a member accessor", "propertySelector");
var propertyInfo = memberExpr.Member as PropertyInfo;
if (propertyInfo == null || propertyInfo.DeclaringType != typeof(TSource))
throw new ArgumentException("must yield a single property on the given object", "propertySelector");
return propertyInfo.Name;
}
The Best Solution
This leaves me with a pretty explicit spec for implementing INotifyPropertyChanged
:
- It will take no more lines than a basic backing field setter.
- It will raise INotifyPropertyChanged and INotifyPropertyChanging.
- It will only raise the properties if the value has changed.
- it will provide an easy way to have internal property changed subscriptions.
- It will not use Lambdas to identify the property.
My solution allows you to do this:
private int _myValue;
public int MyValue
{
get { return _myValue; }
set { SetProperty(ref _myValue, value, "MyValue", OnMyValueChanged, OnMyValueChanging); }
}
private void OnMyValueChanged() { }
private void OnMyValueChanging(int newValue) { }
Isn’t that nice? SetProperty looks like this:
protected void SetProperty(
ref T backingStore, T value,
string propertyName,
Action onChanged = null,
Action onChanging = null)
{
VerifyCallerIsProperty(propertyName);
if (EqualityComparer<T>.Default.Equals(backingStore, value)) return;
if (onChanging != null) onChanging(value);
OnPropertyChanging(propertyName);
backingStore = value;
if (onChanged != null) onChanged();
OnPropertyChanged(propertyName);
}
[Conditional("DEBUG")]
private void VerifyCallerIsProperty(string propertyName)
{
var stackTrace = new StackTrace();
var frame = stackTrace.GetFrames()[2];
var caller = frame.GetMethod();
if (!caller.Name.Equals("set_" + propertyName, StringComparison.InvariantCulture))
throw new InvalidOperationException(
string.Format("Called SetProperty for {0} from {1}", propertyName, caller.Name))
}
The default parameters on SetProperty
allow you to specify the changed callback or the changing callbacks in any permutation with the usual syntax and the stack trace analysis happens at debug time to make sure that the string you provide actually represents the property it’s being called from.
So how do we update dependent properties? The one thing you don’t want to do is ever have external property changing logic directly in your set method. It’s better to call out to a OnMyPropertyChanging
event and have that method update your dependent method. The syntax is a little verbose, but it’s the most readable and extensible way to do it:
private int _foo;
public int Foo
{
get { return _foo; }
set { SetProperty(ref _foo, value, "Foo", OnFooChanged); }
}
private int _bar;
public int Bar
{
get { return _bar; }
set { SetProperty(ref _bar, value, "Bar", OnBarChanged); }
}
private int _foobar;
public int Foobar
{
get { return _foobar; }
private set { SetProperty(ref _foobar, value, "Foobar"); }
}
private void OnFooChanged() { UpdateFoobar(); }
private void OnBarChanged() { UpdateFoobar(); }
private void UpdateFoobar() { Foobar = _foo + _bar; }
In general you should never call OnProeprtyChanged
; that decision is best left to the property and its underlying helper.
I hope this clears the water for some people new to WPF and provides reasons for the veterans to change their tune. If you think I’m wrong, feel free to try and prove it to me in the comments section. After all, duty calls.
The source and XML documentation for all this and a few tests is on github.
05 Dec 2010
When I first started working with WPF professionally, it wasn’t very long before I realized I needed to change the system theme of WPF to give my users a consistent experience across platforms. Not to mention that Vista’s theme was much improved over XP and even more so over the classic theme. Conceptually, this should be feasible, since WPF has its own rendering engine, as opposed to WinForms relying on GDI.
Naturally, the first thing I did was to Google the answer. The first result looked pretty good so I implemented that:
<Application.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="/PresentationFramework.Aero;V4.0.0.0;31bf3856ad364e35;component/themes/aero.normalcolor.xaml" />
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</Application.Resources>
This worked great up until I started styling things. Any time I created a style for one of the system controls, it’d change the underlying style back to the original system them, rather than the one I wanted. It turns out that naturally styles are based on the system theme, rather than implicit style (for reasons we’ll get into later). So the way to base a theme off of the implicit style is like this:
<Style x:Key="GreenButtonStyle" TargetType="Button" BasedOn="{StaticResource {x:Type Button}}">
<Setter Property="Background" Value="Green"/>
<Setter Property="Foreground" Value="White"/>
</Style>
Maybe a little inconvenient, but it gets the job done:
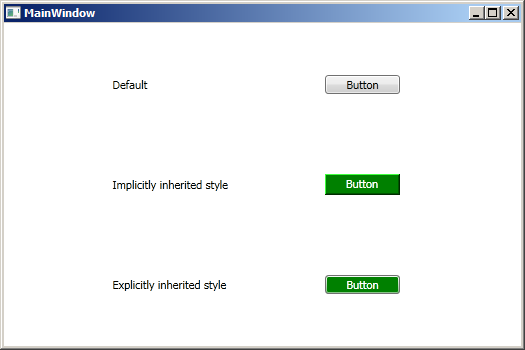
All was well up until I needed to make a new style based off of the default theme. Like many WPF applications, we had some default styles defined to give our controls a clean, consistent look. But in my particular case, I needed to make a whole new visual “branch,” using the default theme as the “trunk.” If I used my BasedOn
workaround, I’d just get my implicit style; if I didn’t I’d get that nasty classic theme.
The more I thought about this, the more I realized that all of these problems were being caused by the fact that I was applying a system theme (aero.normalcolor) as a style on top of the actual system theme, rather than actually changing it. So I set off on a journey in Reflector to find out how WPF picks the current theme. This sounds hard, and it’s actually a lot harder than it sounds. After a dozen or so hours (spread out over a few weeks) and a some guidance by a blog that got really close (unfortunately, my link is now dead), I found out that however WPF calls a native method in uxtheme.dll to get the actual system theme, then stores the result in MS.Win32.UxThemeWrapper
, an internal static class (of course). Furthermore, the properties on the class are read only (and also marked internal), so the best way to change it was by directly manipulating the private fields. My solution looks like this:
public partial class App : Application
{
public App()
{
SetTheme("aero", "normalcolor");
}
/// <summary>
/// Sets the WPF system theme.
/// </summary>
/// <param name="themeName">The name of the theme. (ie "aero")</param>
/// <param name="themeColor">The name of the color. (ie "normalcolor")</param>
public static void SetTheme(string themeName, string themeColor)
{
const BindingFlags staticNonPublic = BindingFlags.Static | BindingFlags.NonPublic;
var presentationFrameworkAsm = Assembly.GetAssembly(typeof(Window));
var themeWrapper = presentationFrameworkAsm.GetType("MS.Win32.UxThemeWrapper");
var isActiveField = themeWrapper.GetField("_isActive", staticNonPublic);
var themeColorField = themeWrapper.GetField("_themeColor", staticNonPublic);
var themeNameField = themeWrapper.GetField("_themeName", staticNonPublic);
// Set this to true so WPF doesn't default to classic.
isActiveField.SetValue(null, true);
themeColorField.SetValue(null, themeColor);
themeNameField.SetValue(null, themeName);
}
}
I call the method in the App
constructor so it sets the values after WPF does its detection, but before the system theme is loaded for rendering.
Here’s what I got back:
Success!
There’s still a couple downsides to this approach. First, I can’t change the theme whenever I want to, and secondly, if the user changes their Windows theme while the application is running, the theme I set gets wiped out.
Wait a minute. If Windows can change the application theme, I can too!
Poking around a little more, I found out that when WPF wants to find a system resource, it also calls System.Windows.SystemResources.EnsureResourceChangeListener()
which makes sure there’s a listener attached to the Windows message pump, ready to interpret events like a theme change.
[SecurityCritical, SecurityTreatAsSafe]
private static void EnsureResourceChangeListener()
{
if (_hwndNotify == null)
{
HwndWrapper wrapper = new HwndWrapper(0, -2013265920, 0, 0, 0, 0, 0, "SystemResourceNotifyWindow", IntPtr.Zero, null);
_hwndNotify = new SecurityCriticalDataClass<HwndWrapper>(wrapper);
_hwndNotify.Value.Dispatcher.ShutdownFinished += new EventHandler(SystemResources.OnShutdownFinished);
_hwndNotifyHook = new HwndWrapperHook(SystemResources.SystemThemeFilterMessage);
_hwndNotify.Value.AddHook(_hwndNotifyHook);
}
}
The handler looks like this:
[SecurityTreatAsSafe, SecurityCritical]
private static IntPtr SystemThemeFilterMessage(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled)
{
WindowMessage message = (WindowMessage) msg;
switch (message)
{
// abridged
case WindowMessage.WM_THEMECHANGED:
SystemColors.InvalidateCache();
SystemParameters.InvalidateCache();
OnThemeChanged();
InvalidateResources(false);
break;
}
return IntPtr.Zero;
}
So the plan was to remove the hook so the message pump couldn’t change the theme out from under me and then call the handler whenever I want. As it turns out, it’s even easier than that. Because the list of hooks is implemented with a WeakReferenceList, all I need to do is null out the private _hwndNotifyHook
field. There’s just one problem here: killing the handler prevents us from receiving system notifications for the other messages like device changes, power updates, etc. So we really need to inject a filter into the pipeline and pass along everything except the ThemeChanged event.
Fair warning: if you get queasy around reflection, you should probably stop reading here.
public static class ThemeHelper
{
private const BindingFlags InstanceNonPublic = BindingFlags.Instance | BindingFlags.NonPublic;
private const BindingFlags StaticNonPublic = BindingFlags.Static | BindingFlags.NonPublic;
private const int ThemeChangedMessage = 0x31a;
private static readonly MethodInfo FilteredSystemThemeFilterMessageMethod =
typeof(ThemeHelper).GetMethod("FilteredSystemThemeFilterMessage", StaticNonPublic);
private static readonly Assembly PresentationFramework =
Assembly.GetAssembly(typeof(Window));
private static readonly Type ThemeWrapper =
PresentationFramework.GetType("MS.Win32.UxThemeWrapper");
private static readonly FieldInfo ThemeWrapper_isActiveField =
ThemeWrapper.GetField("_isActive", StaticNonPublic);
private static readonly FieldInfo ThemeWrapper_themeColorField =
ThemeWrapper.GetField("_themeColor", StaticNonPublic);
private static readonly FieldInfo ThemeWrapper_themeNameField =
ThemeWrapper.GetField("_themeName", StaticNonPublic);
private static readonly Type SystemResources =
PresentationFramework.GetType("System.Windows.SystemResources");
private static readonly FieldInfo SystemResources_hwndNotifyField =
SystemResources.GetField("_hwndNotify", StaticNonPublic);
private static readonly FieldInfo SystemResources_hwndNotifyHookField =
SystemResources.GetField("_hwndNotifyHook", StaticNonPublic);
private static readonly MethodInfo SystemResources_EnsureResourceChangeListener =
SystemResources.GetMethod("EnsureResourceChangeListener", StaticNonPublic);
private static readonly MethodInfo SystemResources_SystemThemeFilterMessageMethod =
SystemResources.GetMethod("SystemThemeFilterMessage", StaticNonPublic);
private static readonly Assembly WindowsBase =
Assembly.GetAssembly(typeof(DependencyObject));
private static readonly Type HwndWrapperHook =
WindowsBase.GetType("MS.Win32.HwndWrapperHook");
private static readonly Type HwndWrapper =
WindowsBase.GetType("MS.Win32.HwndWrapper");
private static readonly MethodInfo HwndWrapper_AddHookMethod =
HwndWrapper.GetMethod("AddHook");
private static readonly Type SecurityCriticalDataClass =
WindowsBase.GetType("MS.Internal.SecurityCriticalDataClass`1")
.MakeGenericType(HwndWrapper);
private static readonly PropertyInfo SecurityCriticalDataClass_ValueProperty =
SecurityCriticalDataClass.GetProperty("Value", InstanceNonPublic);
/// <summary>
/// Sets the WPF system theme.
/// </summary>
/// <param name="themeName">The name of the theme. (ie "aero")</param>
/// <param name="themeColor">The name of the color. (ie "normalcolor")</param>
public static void SetTheme(string themeName, string themeColor)
{
SetHwndNotifyHook(FilteredSystemThemeFilterMessageMethod);
// Call the system message handler with ThemeChanged so it
// will clear the theme dictionary caches.
InvokeSystemThemeFilterMessage(IntPtr.Zero, ThemeChangedMessage, IntPtr.Zero, IntPtr.Zero, false);
// Need this to make sure WPF doesn't default to classic.
ThemeWrapper_isActiveField.SetValue(null, true);
ThemeWrapper_themeColorField.SetValue(null, themeColor);
ThemeWrapper_themeNameField.SetValue(null, themeName);
}
public static void Reset()
{
SetHwndNotifyHook(SystemResources_SystemThemeFilterMessageMethod);
InvokeSystemThemeFilterMessage(IntPtr.Zero, ThemeChangedMessage, IntPtr.Zero, IntPtr.Zero, false);
}
private static void SetHwndNotifyHook(MethodInfo method)
{
var hookDelegate = Delegate.CreateDelegate(HwndWrapperHook, FilteredSystemThemeFilterMessageMethod);
// Note that because the HwndwWrapper uses a WeakReference list, we don't need
// to remove the old value. Simply killing the reference is good enough.
SystemResources_hwndNotifyHookField.SetValue(null, hookDelegate);
// Make sure _hwndNotify is set!
SystemResources_EnsureResourceChangeListener.Invoke(null, null);
// this does SystemResources._hwndNotify.Value.AddHook(hookDelegate)
var hwndNotify = SystemResources_hwndNotifyField.GetValue(null);
var hwndNotifyValue = SecurityCriticalDataClass_ValueProperty.GetValue(hwndNotify, null);
HwndWrapper_AddHookMethod.Invoke(hwndNotifyValue, new object[] { hookDelegate });
}
private static IntPtr InvokeSystemThemeFilterMessage(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, bool handled)
{
return (IntPtr)SystemResources_SystemThemeFilterMessageMethod.Invoke(null, new object[] { hwnd, msg, wParam, lParam, handled });
}
private static IntPtr FilteredSystemThemeFilterMessage(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled)
{
if (msg == ThemeChangedMessage)
return IntPtr.Zero;
return InvokeSystemThemeFilterMessage(hwnd, msg, wParam, lParam, handled);
}
}
What a mess! What’s really annoying is that this entire process could have (and, I argue, should have) been made public to user code. In the very least, I’d like to be able to decorate my assembly with an attribute declaring which theme I’d like to run under, but I think it’s completely reasonable to have an public static class provide the same API I have here.
The whole test suite is available here on GitHub.
27 Sep 2010
Last week I interviewed colleague Kalani Thielen, Lab49’s resident expert on programming language theory. We discussed some of the new languages we’ve seen this decade, the recent functional additions to imperative languages, and the role DSLs will play in the future. Read on for the full interview.
DM F#, Clojure, and Scala are all fairly new and popular languages this decade, the former two with striking resemblance to OCaml and Lisp, respectively, and the lattermost being more original in syntax. In what way do these languages represent forward thinking in language design, or how do they fail to build upon lessons learned in more venerable languages like Haskell?
KT It’s a common story in the world of software that time brings increasingly precise and accurate programs. Anybody who grew up playing games on an Atari 2600 has witnessed this firsthand. The image of a character has changed from one block, to five blocks, to fifty blocks, to (eventually) thousands of polygons. By this modern analog of the Greeks’ method of exhaustion, Mario’s facial structure has become increasingly clear. This inevitable progression, squeezed out between the increasing sophistication of programmers and the decreasing punishment of Moore’s Law, has primarily operated at three levels: the images produced by game programs, the logic of the game programs themselves, and finally the programming languages with which programs are produced. It’s this last group that deserves special attention today.
The lambda calculus (and its myriad derivatives) exemplifies this progression at the level of programming languages. In the broadest terms, you have the untyped lambda calculus at the least-defined end (which closely fits languages like Lisp, Scheme, Clojure, Ruby and Python), and the calculus of constructions at the most-defined end (which closely fits languages like Cayenne and Epigram). With the least imposed structure, you can’t solve the halting problem, and with the most imposed structure you (trivially) can solve it. With the language of the untyped lambda calculus, you get a blocky, imprecise image of what your program does, and in the calculus of constructions you get a crisp, precise image.
Languages like F#, Scala and Haskell each fall somewhere in between these two extremes. None of them are precise enough to accept only halting programs (although Haskell is inching more and more toward a dependently-typed language every year). Yet all of them are more precise than (say) Scheme, where fallible human convention alone determines whether or not the “+” function returns an integer or a chicken. But even between these languages there is a gulf of expressiveness. Where a language like C is monomorphically-typed (and an “int_list” must remain ever distinguished from a “char_list”), F# introduces principled polymorphic types (where, pun intended, you can have “’a list” for any type ‘a). Beyond that, Haskell (and Scala) offer bounded polymorphism so that constraints can be imposed (or inferred) on any polymorphic type – you can correctly identify the “==” function as having type “Eq a => a -> a -> Bool” so that equality can be determined only on those types which have an equivalence relation, whereas F# has to make do with the imprecise claim that its “=” function can compare any types.
No modern programming language is perfect, but the problems that we face today and the history of our industry points the way toward ever more precise languages. Logic, unreasonably effective in the field of computer science, has already set the ideal that we’re working toward. Although today you might hear talk that referential transparency in functional languages makes a parallel map safe, tomorrow it will be that a type-level proof of associativity makes a parallel reduce safe. Until then, it’s worth learning Haskell (or Scala if you must), where you can count the metaphorical fingers on Mario’s hands, though not yet the hairs of his moustache.
DM One might assume that increased “precision” in a language would come at the cost of increased complexity in concepts and/or syntax. In a research scenario, the ability to solve the halting problem certainly has its merits, but is that useful for modern commercial application development, and, if so, does it justify the steeper learning curve?
KT It’s absolutely true that languages that can express concepts more precisely also impose a burden on programmers, and that a major part of work in language design goes into making that burden as light as possible (hence type-inference, auto roll/unroll for recursive types, pack/unpack for existential types, etc).
However — to your point about the value of that increased precision – I would argue that it’s even more important in commercial application development than in academia. For example, say you’ve just rolled out a new pricing server for a major client. Does it halt? There’s a lot more riding on that answer than you’re likely to find in academia. And really, whether or not it halts is just one of the simplest questions you can ask. What are its time/space characteristics? Can it safely be run in parallel? Is it monotonic? In our business, these questions translate into dollars and reputation. Frankly I think it’s amazing that we’ve managed to go on this long without formal verification.
DM Most dynamic languages have some air of functional programming to them, while still being fundamentally imperative. Even more rigorous imperative languages like C# are picking up on a more functional style of programming. The two languages you mentioned earlier, Cayenne and Epigram, are both functional languages. Are we moving toward a pure functional paradigm, or will there continue to be a need for imperative/functional hybrids?
KT What is a “functional language”? If it’s a language with first-class functions, then C is a functional language. If it’s a language that disallows hidden side-effects, then Haskell isn’t a functional language. I think that, at least as far as discussing the design and development of programming languages is concerned, it’s well worth getting past that sort of “sales pitch”.
I believe that we’re moving toward programming languages that allow programmers to be increasingly precise about what their programs are supposed to do, up to the level of detail necessary. The great thing about referential transparency is that it makes it very easy to reason about what a function does — it’s almost as easy as high school algebra. However, if you’re not engaged in computational geometry, but rather need to transfer some files via FTP, there’s just no way around it. You’ve got to do this, then this, then this, and you’ll need to switch to something more complicated, like Hoare logic, to reason about what your program is doing. Even there, you have plenty of opportunity for precision — you expect to use hidden side-effects but only of a certain type (transfer files but don’t launch missiles).
But maybe the most important tool in programming is logic itself. It’s a fundamental fact – well known in some circles as the “Curry-Howard isomorphism” – that programs and proofs are equivalent in a very subtle and profound way. This fact has produced great wealth for CS researchers, who can take the results painstakingly derived by logicians 100 years ago and (almost mechanically) publish an outline of their computational analog. Yet, although this amazing synthesis has taken place rivaling the unification of electricity and magnetism, most programmers in industry are scarcely aware of it. It’s going to take some time.
I think there’s a good answer to your question in that correspondence between logic and programming. The function, or “implication connective” (aka “->”), is an important tool and ought to feature in any modern language. As well, there are other logical connectives that should be examined. For example, conjunction is common (manifested as pair, tuple, or record types in a programming language), but disjunction (corresponding to variant types) is less common though no less important. Negation (corresponding to continuations consuming the negated type), predicated quantified types, and so on. The tools for building better software are there, but we need to work at recognizing them and putting them to good use.
Anybody interested in understanding these logical tools better should pick up a copy of Benjamin Pierce’s book Types and Programming Languages.
DM Many frameworks have a one or more DSLs to express things as mundane as configuration to tasks as complicated as UI layout, in an effort to be more express specific kinds of ideas more concisely. Do you see this as an expanding part of the strategy for language designers to increase expressiveness in code? Is it possible that what we consider “general purpose languages” today will become more focused on marshalling data from one DSL to another, or will DSLs continue to remain a more niche tool?
KT I guess it depends on what you mean by “DSL”. Like you say, some people just have in mind some convenient shorthand serialization for data structures (I’ve heard some people refer to a text format for orders as a DSL, for example). I’m sure that will always be around, and there’s nothing really profound about it.
On the other hand, by “DSL” you could mean some sub-Turing language with non-trivial semantics. For example, context-free grammars or makefiles. Modern programming languages, like Haskell, are often used to embed these “sub-languages” as combinator libraries (the “Composing Contracts” paper by Simon Peyton-Jones et al is a good example of this). I think it’s likely that these will continue as valuable niche tools. If you take monadic parser combinators for example, it’s very attractive the way that they fit together within the normal semantics of Haskell, however you’ve got to go through some severe mental gymnastics to determine for certain what the space/time characteristics of a given parser will be. Contrast that with good old LALR(1) parsers, where if the LR table can be derived you know for certain what the space/time characteristics of your parser will be.
On the third hand, if a “DSL” is a data structure with semantics of any kind, your description of a future where programs are written as transformations between DSLs could reasonably describe the way that compilers are written today. Generally a compiler is just a sequence of semantics-preserving transformations between data structures (up to assembly statements). I happen to think that’s a great way to write software, so I hope it’s the case that it will become ever more successful.